20. Medium access control
org.arl.unet.Services.MAC
20.1. Overview
Agents offering the medium access control (MAC) service advise other agents on when they may be permitted to make transmissions, in an effort to reduce collisions and improve network throughput.
MAC protocols that use PDUs for channel reservation may support piggybacking of client data in the PDU. If such support is available, it is advertised using a non-zero
reservationPayloadSize
parameter. A
ReservationReq
should provide the payload data to be sent to a peer node (as part of RTS or equivalent PDU) to whom the reservation is made. If that node wishes to send payload data back (as part of CTS or equivalent PDU), it may send a
ReservationAcceptReq
in response to a
ReservationStatusNtf
to provide its payload data.
Protocol data units (PDUs) are protocol-specific datagrams exchanged by nodes in a network. MAC protocls that use a handshake often use three basic type of PDUs — request to send (RTS), clear to send (CTS) and acknowledgement (ACK). |
20.1.1. Messages
Agents supporting the MAC service provide messages to request, grant and cancel reservations:
-
ReservationReq
⇒ReservationRsp
/REFUSE
— request a reservation -
ReservationCancelReq
⇒AGREE
/REFUSE
— cancel a reservation -
ReservationAcceptReq
⇒AGREE
/REFUSE
— request piggybacking of payload in a reservation PDU, typically sent by a client on receiving aReservationStatusNtf[status: REQUEST]
notification -
TxAckReq
⇒AGREE
/REFUSE
— request transmission of acknowledgement payload -
ReservationStatusNtf
— sent to requestor or agent’s topic when a reservation-related events occur
While we use the term reservation for all MAC agents, it is important to understand that MAC agents are typically unable to guarantee that no other nodes transmit during the reservation period. The reservation is on a best-effort basis, and the probability of collision is dependent on the underlying MAC protocol in use. For example, a handshake-based MAC (using RTS/CTS) might be able to inform other nodes' MAC agents about a reservation, while carrier-sensing MAC agents may rely on hearing the transmission from another node to backoff reservations at their node. A MAC agent must, however, guarantee that no two agents on the same node are granted reservation at the same time.
Agents providing the MAC service are able to queue reservation requests from multiple agents, and grant reservations in order of arrival of request (or priority, or some other measure of fairness). It is, however, recommended that one agent only make one request to the MAC at a time, and submit its next request after the previous reservation is completed. An agent providing the MAC service may choose to refuse reservation requests from an agent that has pending reservation requests already in the queue.
20.1.2. Parameters
Agents offering the MAC service support the following parameters:
-
channelBusy
— true if channel is busy, false otherwise -
reservationPayloadSize
— maximum size of payload (bytes) that can be piggybacked in a reservation PDU -
ackPayloadSize
— maximum size of acknowledgement (bytes) that can be included in an ACK PDU -
maxReservationDuration
— maximum duration of reservation in seconds -
recommendedReservationDuration
— recommended duration of reservation in seconds (null, if unspecified)
A MAC agent advertises the maximum supported reservation duration (
maxReservationDuration
), and must honor reservation requests for up to this duration. However, the network performance might suffer if all agents use very long reservations. To address this, the MAC agent also advertises a shorter recommendation for reservation duration (
recommendedReservationDuration
). Most agents should request reservations of this duration (or shorter) unless unavoidable.
20.1.3. Capabilities
Agents may support several optional capabilities:
An agent advertising this capability must be able to send acknowlegements as part of the MAC protocol. The agent must support the
TxAckReq
request to provide acknowledgement payload to be transmitted to the peer node at the end of the reservation. On reception, this payload should be delivered in the
ReservationStatusNtf[status: END]
on the peer node.
Agents advertising this capability must honor priority settings in the reservation request.
Agents advertising this capability must honor time-to-live settings in the reservation request.
Agents advertising this capability must support scheduling of reservations in the future, through the use of the
startTime
attribute of the
ReservationReq
.
20.2. Basic MAC functionality
MAC agents advise other agents that wish to transmit (we shall call them clients ) on when they may do so. MAC agents make channel reservations on behalf of their clients, as necessary. Some MAC protocols such as Aloha and TDMA may not require explicit handshake for reservation, while others such as MACA and FAMA may involve control packet exchanges between peer MAC agents on various nodes. In either case, a typical client with data to transmit starts by asking the prevailing MAC agent for a channel reservation:
// client wishes to transmit data to "destination" for specified "duration"
def mac = agentForService(Services.MAC)
if (mac) {
def req = new ReservationReq(recipient: mac, to: destination, duration: duration) (1)
def rsp = request(req)
if (rsp && rsp.performative == Performative.AGREE) {
def ntf = receive(ReservationStatusNtf, timeout) (2)
if (ntf && ntf.inReplyTo == req.messageID && ntf.status == ReservationStatus.START) {
// :
// transmit data for requested duration
// :
}
}
}
1 | Send a channel reservation request. |
2 | Wait for a channel reservation notification. |
In the above sample code, error handling has been omitted for simplicity. In reality, you would want to have else clauses to handle reservation failures. The MAC agent not only sends a
ReservationStatusNtf[status: START]
notification, but also a
ReservationStatusNtf[status: END]
notification at the end of the reservation duration. The sample code above ignores this notification, but a well-behaved client should ensure that the transmission does not exceed the requested duration.
20.3. Working with MAC payloads
Messages such as
ReservationReq
and
ReservationStatusNtf
may carry payloads, when the MAC protocol supports them. When payloads are supported, additional messages such as
ReservationAcceptReq
,
TxAckReq
and
TxAckNtf
are available for clients to provide payloads to the MAC service provider to piggyback on the MAC PDUs. A typical exchange is illustrated in
Figure 9
.
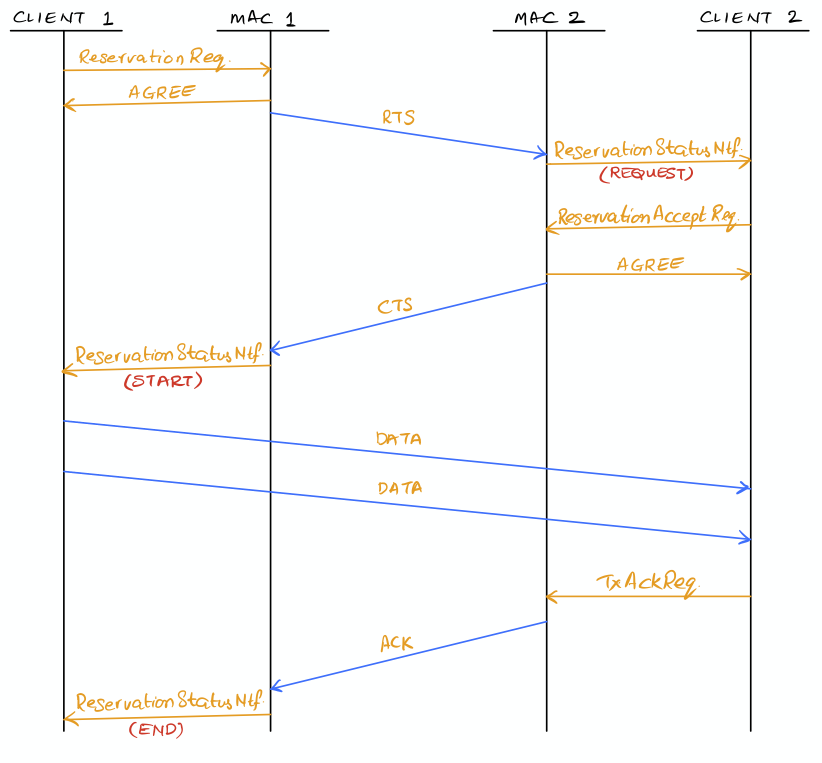
For a MAC reservation initiated by node A with node B, we elaborate on the steps for a full reservation lifecycle with payloads:
-
On node A, the client (agent) sends a
ReservationReq
to the MAC (agent), with an optional payload. The MAC accepts the request. -
MAC on node A sends an RTS PDU with the payload to the MAC on node B.
-
MAC on node B generates a
ReservationStatusNtf[status: REQUEST]
message and publishes it on its topic. A client subscribing to this topic receives the notification. -
If the client on node B wants to send back some payload with the CTS PDU, it immediately sends a
ReservationAcceptReq
to the MAC, with the payload. -
The MAC accepts the request and responds to node A’s MAC with a clients PDU containing the payload.
-
The payload is delivered to the client on node A as part of a
ReservationStatusNtf[status: START]
message marking the start of the reservation time. -
During the reservation, the two nodes exchange data as they wish.
-
If the client on node B wishes to provide an acknowledgment (with a payload), it sends a
TxAckReq
message before the reservation duration ends, and the MAC on node B accepts. -
The MAC on node B sends an ACK PDU with the payload to the MAC on node A. The ACK PDU marks the end of the channel reservation. The MAC delivers this acknowledgment payload to the client on node A as a part of the
ReservationStatusNtf[status: END]
message. -
If node B does not send an ACK PDU, when the channel reservation ends, the MAC on node A sends a
ReservationStatusNtf[status: END]
message to its client.
20.4. Examples
Sample MAC implementations are illustrated in Chapter 29 .
<<< [Address resolution] | [Single-hop links] >>> |